Handling User Input Safely: The Foundation of Secure Web Applications
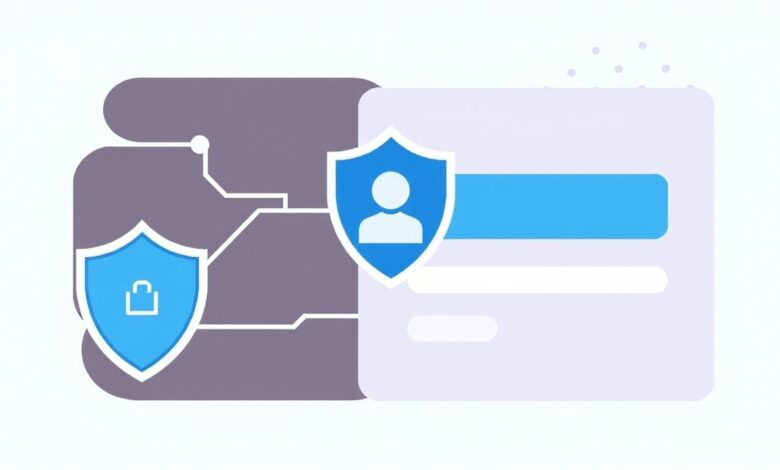
In the world of web development, user input is both essential and potentially dangerous. Every form field, URL parameter, or API endpoint that accepts data from a user is a potential gateway for attackers. Failing in handling user input safely is akin to leaving your front door wide open. It’s a fundamental aspect of secure coding, yet overlooking it leads to some of the most common and damaging vulnerabilities, such as SQL injection and Cross-Site Scripting (XSS). This post dives into the core principles and best practices for securely managing user-submitted data.
Why Handling User Input Safely is Non-Negotiable
User input can come in various forms – text fields, dropdowns, file uploads, hidden fields, cookies, and more. Attackers exploit these inputs by injecting malicious code or unexpected data formats. According to recent web security reports, injection flaws consistently rank among the top web application security risks. Failing to handle input correctly can lead to:
- Data breaches (theft of sensitive user information)
- Website defacement
- Server compromise
- Loss of user trust and reputation damage
- Financial losses and legal liabilities
Therefore, implementing robust input handling mechanisms isn’t just a ‘nice-to-have’; it’s a critical requirement for any application.
The Core Strategy: Validate → Sanitize → Secure
A defense-in-depth approach is crucial. Relying on a single technique is insufficient. The mantra should be to validate input early, sanitize it appropriately, and handle it securely throughout its lifecycle, especially during database interactions and output rendering.
1. Validate Early and Strictly
Validation is the process of checking if the user input conforms to expected rules – format, type, length, and range. The OWASP Input Validation Cheat Sheet emphasizes validating input as early as possible in the data flow. Why?
- Detect Malice Early: It helps catch obviously malformed or malicious data before it penetrates deeper into your application logic.
- Enforce Business Rules: Ensures data meets application requirements (e.g., an email address looks like an email address, a phone number contains only digits and specific symbols, a quantity is within a valid range).
- Reduce Attack Surface: By rejecting invalid data immediately, you minimize the chances of it reaching vulnerable code paths.
Best Practices for Validation:
- Use Whitelisting (Allowlisting): Define exactly what *is* allowed, rather than trying to block known bad inputs (blacklisting). Blacklists are notoriously difficult to maintain and easily bypassed. For example, if a field expects a 5-digit zip code, validate that it contains exactly five digits (`^[0-9]{5}$`) rather than trying to list all possible malicious strings.
- Validate on Both Client and Server: Client-side validation (using JavaScript) provides quick feedback to the user but can be easily bypassed. Server-side validation is non-negotiable as it acts as the authoritative check.
- Check Data Types, Lengths, Formats, and Ranges: Be specific. If you expect an integer, ensure it’s an integer. If a username has a maximum length, enforce it. Use regular expressions carefully for format validation.
[Hint: Insert image illustrating the concept of whitelisting vs. blacklisting here]
2. Sanitize for Specific Contexts
Sanitization involves cleaning or modifying user input to remove potentially harmful characters or sequences before using it in a particular context (like embedding in HTML or using in a database query). It’s crucial because even valid data (according to format rules) might contain characters that are dangerous in certain contexts.
Key Sanitization Areas:
- Preventing Cross-Site Scripting (XSS): When displaying user input back in an HTML page, characters like `<`, `>`, `&`, `’`, and `”` can be used to inject malicious scripts. Sanitization for HTML context (often called HTML escaping or encoding) converts these characters into their harmless HTML entity equivalents (e.g., `<` becomes `<`). Never render raw user input directly into your HTML/JavaScript.
- Preventing SQL Injection (SQLi): When using user input to build database queries, special characters like single quotes (`’`), double quotes (`”`), semicolons (`;`), and comments (`–`, `/*`) can be used to alter the query’s logic, potentially allowing attackers to steal or modify data. The best defense here is not just sanitization but using parameterized queries (prepared statements).
Many modern web frameworks provide built-in functions for sanitization and output encoding. Leverage them!
3. Secure Database Interactions
Storing and retrieving user input from databases requires careful handling. Blindly trusting data, even if previously validated, is risky.
- Use Parameterized Queries (Prepared Statements): This is the single most effective way to prevent SQL injection. The database driver treats the user input strictly as data, not executable code, regardless of what characters it contains.
- Least Privilege Principle: Ensure the database user account your application uses has only the minimum necessary permissions. It shouldn’t have permissions to drop tables if it only needs to read and write specific data.
- Escape When Parameterization Isn’t Possible: In rare cases where parameterized queries can’t be used, meticulously escape all user-supplied input using a database-specific escaping function before incorporating it into a query. This is less secure and harder to get right than parameterization.
[Hint: Insert image/diagram showing how parameterized queries prevent SQL injection here]
4. Don’t Forget Output Encoding
As mentioned under sanitization, safely rendering data back to the user is critical. The context matters. Data safe for HTML might not be safe for JavaScript, CSS, or a URL.
- Contextual Output Encoding: Apply the correct encoding based on where the data will be placed. HTML entity encoding for HTML body, attribute encoding for HTML attributes, JavaScript encoding for JavaScript data blocks, URL encoding for URL components, etc.
- Leverage Frameworks: Modern templating engines and frameworks (like React, Angular, Vue, Vaadin, Django, Rails) often handle contextual output encoding automatically or provide secure mechanisms to do so. Understand how your framework handles this.
Embrace Secure Coding Principles
Handling user input safely is part of a broader secure coding mindset.
- Defense in Depth: Use multiple layers of security (validation, sanitization, parameterized queries, output encoding).
- Fail Securely: If validation fails, reject the input gracefully without leaking system information.
- Keep Libraries/Frameworks Updated: Security vulnerabilities are often found and patched in frameworks and libraries. Stay current.
- Regular Security Testing: Use security scanners and perform code reviews to identify input handling flaws.
Handling user input safely is not a one-time task but an ongoing process. By consistently applying validation, sanitization, parameterized queries, and contextual output encoding, developers can significantly reduce the risk of common web vulnerabilities and build more robust, trustworthy applications. For more in-depth strategies, consider exploring resources like the Secure Coding Principles guide.